Method-1
---------
Swapping of two numbers using third variable
import java.util.Scanner;
class SwapNumbers
{
public static void main(String args[])
{
int x, y, temp;
System.out.println("Enter x and y");
Scanner in = new Scanner(System.in);
x = in.nextInt();
y = in.nextInt();
System.out.println("Before Swapping\nx = "+x+"\ny = "+y);
temp = x;
x = y;
y = temp;
System.out.println("After Swapping\nx = "+x+"\ny = "+y);
}
}
Method-2
-----------
Swapping of two numbers without using third variable
import java.util.Scanner;
class SwapNumbers
{
public static void main(String args[])
{
int x, y;
System.out.println("Enter x and y");
Scanner in = new Scanner(System.in);
x = in.nextInt();
y = in.nextInt();
System.out.println("Before Swapping\nx = "+x+"\ny = "+y);
x = x + y;
y = x - y;
x = x - y;
System.out.println("After Swapping\nx = "+x+"\ny = "+y);
}
}
Mehod-3
------------
Swapping of two numbers using XOR Operator
int a = 2; //0010 in binaryint b = 4; //0100 in binarya = a^b; //now a is 6 and b is 4b = a^b; //now a is 6 but b is 2 (original value of a)a = a^b; //now a is 4 and b is 2, numbers are swappedMethod-4------------
Swapping Of Two Numbers Using Multiplication and division operatorsint a = 6;int b = 3;//swapping value of two numbers without using temp variable using multiplication and divisiona = a*b; //now a is 18 and b is 3b = a/b; //now a is 18 but b is 6 (original value of a)a = a/b; //now a is 3 and b is 6, numbers are swappedMethod-5---------Swapping of two numbers in a single statementint a = 10; int b = 20; a = ( a + b ) - ( b = a ); Method-6------------Swapping of two numbers in a single statementint a = 10; int b = 20;a = b + 0 * (b = a);Method-6
-----------
import java.io.*;
class swap{
public static void main(String args[]) throws IOException{
BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
System.out.print("Enter 1st no: ");
int x=Integer.parseInt(br.readLine());
System.out.print("Enter 2nd no: ");
int y=Integer.parseInt(br.readLine());
System.out.println("\nNumbers Before Swapping:");
System.out.println("x = "+x);
System.out.println("y = "+y);
//Swapping Method 2
x = x^y;
y = x^y;
x = x^y;
System.out.println("\nNumbers After Swapping:");
System.out.println("x = "+x);
System.out.println("y = "+y);
}
}
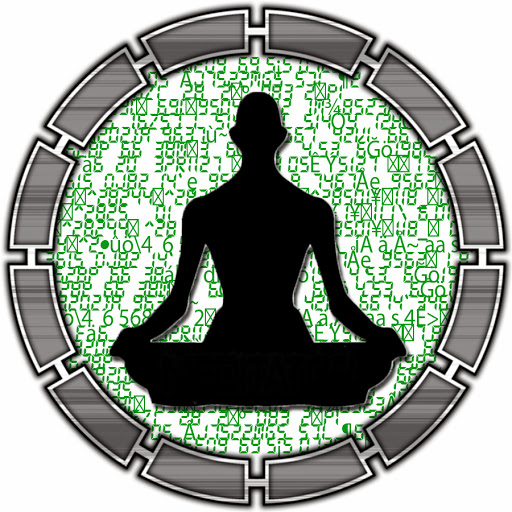
This post was written by: Author Name
Your description comes here!
Get Free Email Updates to your Inbox!
on Monday, 14 April 2014
Post a Comment